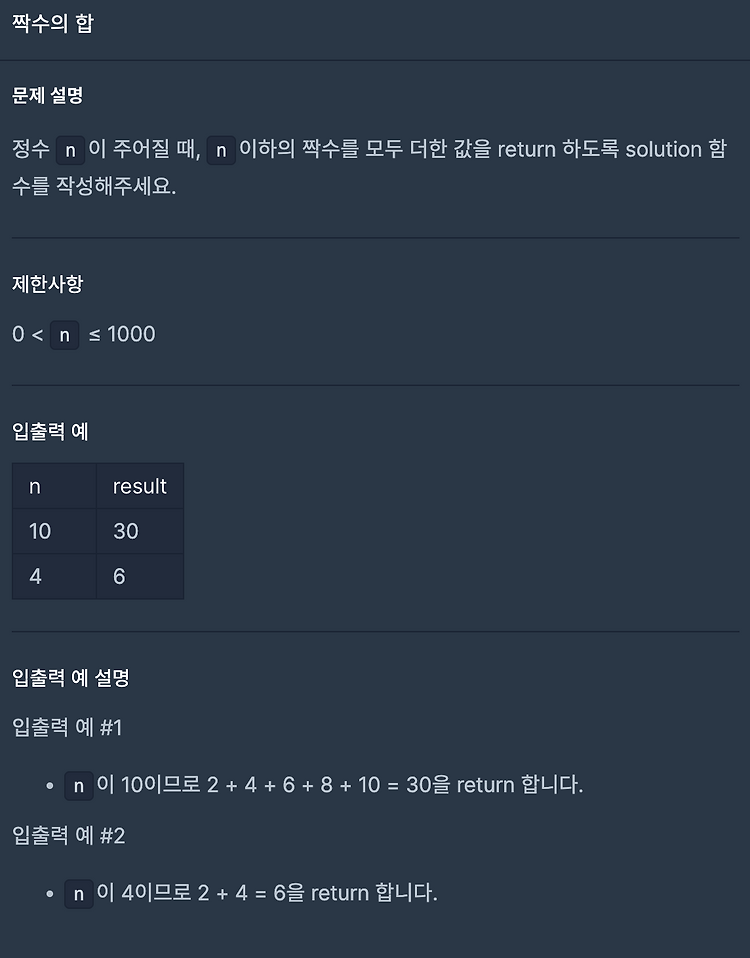
[알고리즘] 짝수의 합 Java
import java.util.stream.IntStream; class Solution { public int solution(int n) { return IntStream.rangeClosed(1, n).filter(i -> i % 2 == 0).sum(); } }
import java.util.stream.IntStream; class Solution { public int solution(int n) { return IntStream.rangeClosed(1, n).filter(i -> i % 2 == 0).sum(); } }
class Solution { public int solution(int hp) { int answer = 0; while (hp != 0) { answer += hp / 5; hp = hp % 5; answer += hp / 3; hp = hp % 3; answer += hp / 1; hp = hp % 1; } return answer; } }
import java.util.HashMap; import java.util.Map; class Solution { public String solution(String rsp) { String answer = ""; Map rspMap = new HashMap(){ { put("2", "0"); put("0", "5"); put("5", "2"); } }; String[] split = rsp.split(""); for (String s : split) { answer += rspMap.get(s); } return answer; } }
import java.util.HashMap; import java.util.Map; class Solution { public String solution(String letter) { String answer = ""; Map morse = new HashMap(); morse.put(".-", "a"); morse.put("-...", "b"); morse.put("-.-.", "c"); morse.put("-..", "d"); morse.put(".", "e"); morse.put("..-.", "f"); morse.put("--.", "g"); morse.put("....", "h"); morse.put("..", "i"); morse.put(".---", "j"); morse.put("-.-"..
import java.util.Arrays; import java.util.stream.Collectors; class Solution { public String solution(String my_string, int n) { return Arrays.asList(my_string.split("")).stream() .map(s -> s.repeat(n)) .collect(Collectors.joining()); } } Java String repeat() 메서드는 값이 주어진 횟수만큼 이 문자열을 연결한 새 문자열을 반환합니다 Java String repeat()은 문자열을 특정 횟수만큼 연결하는 데 사용할 수 있는 간단한 유틸리티 메서드입니다. 결과 문자열 길이가 너무 크면 OutOfMemoryEr..
class Solution { public int[] solution(int numer1, int denom1, int numer2, int denom2) { int[] answer = new int[2]; int numerator = numer1 * denom2 + denom1 * numer2; int denominator = denom1 * denom2; int mod = gcd(numerator, denominator); answer[0] = numerator / mod; answer[1] = denominator / mod; return answer; } // 유클리드 호제법 public static int gcd(int a, int b) { // a가 b보다 큰 경우에 대해서 유클리드 호제법 수..
class Solution { public int solution(int n) { return lcm(n, 6) / 6; } static int gdc(int a, int b) { if (a < b) { int temp = a; a = b; b = temp; } while (b != 0) { int r = a % b; a = b; b = r; } return a; } static int lcm(int a, int b) { return a * b / gdc(a, b); } }
import java.util.Arrays; class Solution { public int solution(int[] array, int n) { return (int) Arrays.stream(array).filter(i -> i == n).count(); } }